WebRTC is a streaming protocol with real-time communication (RTC) capabilities in web browsers and mobile applications. WebRTC allows for audio, video, and data sharing between browser clients (or native apps) without needing plugins or any third-party software. It uses JavaScript APIs to establish peer-to-peer communication, making it highly suitable for applications like video calls, file sharing, live streaming, and instant messaging.
WebRTC testing is challenging because it requires real-time communication, multiple browsers, and varying network conditions. Selenium can help by automating browser actions and testing WebRTC features, and other tools can simulate network conditions for more in-depth testing. Let us learn more about selenium.
Selenium is an extremely useful open-source tool for automating web browsers. It allows developers and testers to interact with web applications using scripts, which simulate user actions such as clicking buttons, filling out forms, and navigating between pages. Selenium supports a variety of programming languages (including Python, Java, and JavaScript) and works with most major browsers.
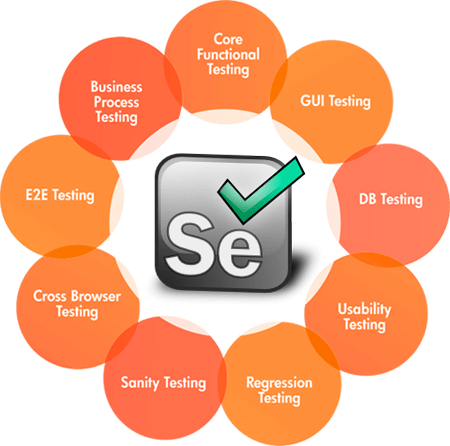
There are numerous components in selenium. Selenium WebDriver, Selenium Grid, and Selenium IDE are all designed to meet different testing needs. These components play a unique role in the automation process, helping teams streamline their testing efforts.
Overview of the Selenium Components
1. Selenium WebDriver
- A core component that directly controls the browser by sending commands and retrieving results.
- Allows you to write test scripts in programming languages like Python, Java, or JavaScript.
- Supports most modern browsers (e.g., Chrome, Firefox, Edge).
- Ideal for creating flexible, real-time browser automation for dynamic web apps.
2. Selenium IDE (Integrated Development Environment)
- A browser extension (available for Chrome and Firefox) used for recording and playback of tests.
- Useful for beginners and quick prototyping as it doesn’t require programming knowledge.
- Limited in complexity compared to WebDriver, making it suitable for simple test cases.
3. Selenium Grid
- Enables parallel testing by distributing test execution across multiple machines and browsers.
- Ideal for cross-browser and cross-platform testing at scale.
- Works by setting up a Hub (central controller) and multiple Nodes (machines running tests).
How WebRTC Testing Works with Selenium:
In order to test WebRTC with Selenium, the browser interactions necessary for real-time communication (such as video calls and live streaming) must be automated. WebRTC presents challenges because it relies on peer-to-peer connections, live audio/video streams, and dynamic network conditions.
- Accessing WebRTC Pages: Selenium can navigate to a WebRTC-enabled web page and simulate user actions to initiate or join a call.
- Simulating Media: By using virtual devices (e.g., fake webcams and microphones), Selenium can interact with WebRTC applications without requiring real hardware.
- Validating Peer Connections: Selenium scripts can verify if the WebRTC session starts by checking browser console logs,
getStats()
API data, or DOM elements. - Testing Scenarios:
- Ensure audio/video streams are connected.
- Validate the stability of peer-to-peer connections under different conditions.
- Check for proper handling of user permissions (camera, microphone).
- Performance Monitoring: Capture and analyze WebRTC stats (e.g., latency, bitrate, packet loss) via JavaScript execution in the browser.
Moving further, we will learn the installation of the selenium driver and how to use it to do the WebRTC testing with Ant Media Server.
Installation of Selenium WebDriver
To get started with Selenium, you first need to set up your environment. We will use Chrome browser, Chrome driver, and Selenium on the Ubuntu system.
- Google Chrome: Download and install the latest version of Chrome from the below Linux commands.
wget https://dl.google.com/linux/direct/google-chrome-stable_current_amd64.deb
sudo dpkg -i google-chrome-stable_current_amd64.deb
- ChromeDriver: Ensure you download a version of ChromeDriver that matches your version of Chrome from the ChromeDriver download page. This is essential as it enables Selenium to communicate with your browser.
For example, if the version is 131.0.6778.108, then use the below commands to install the driver for that version.
wget https://storage.googleapis.com/chrome-for-testing-public/131.0.6778.69/linux64/chromedriver-linux64.zip
unzip chromedriver-linux64.zip
cd chromedriver-linux64
sudo mv chromedriver /usr/bin/chromedriver
sudo chown root:root /usr/bin/chromedriver
sudo chmod +x /usr/bin/chromedriver
- Selenium Python Bindings: These allow you to control your browser directly from Python. You can download them from the Selenium downloads page.
You can also download the dependencies using the below Python commands.
sudo apt install python3
sudo apt update
sudo apt install python3-pip
pip install selenium
pip install requests
Python Script for WebRTC Testing
In this section, we will use Python and Selenium to write a basic sample script for WebRTC testing. For testing, we use Ant Media Server’s WebRTC publishing sample page.
# Import Dependencies
import os
import time
import requests
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.chrome.options import Options
# Chrome headless mode
options = Options()
options.add_argument('--headless')
options.add_argument("--window-size=1920,1080");
options.add_argument("--use-fake-ui-for-media-stream")
options.add_argument("--use-fake-device-for-media-stream")
driver = webdriver.Chrome(options=options)
driver.maximize_window()
# Testing WebRTC Sample Page
try:
driver.execute_script("window.open('https://test.antmedia.io:5443/24x7test?id=test', '_blank');")
driver.switch_to.window(driver.window_handles[1])
time.sleep(10)
driver.switch_to.frame(0)
time.sleep(3)
driver.find_element(By.XPATH, "/html/body/div/div/div[8]/button[1]").click()
time.sleep(10)
driver.find_element(By.XPATH, "/html/body/div/div/div[7]/div[1]/a").click()
time.sleep(10)
driver.find_element(By.XPATH, "/html/body/div/div/div[8]/button[2]").click()
time.sleep(3)
print("WebRTC to WebRTC is successful")
except:
print("WebRTC publishing test is failed")
driver.quit()
In the above code, we imported the dependencies, initialized the Chrome browser in headless mode, and published the WebRTC stream by opening the page in that browser. For more reference about selenium and its usage, check out this blogpost.
The above WebRTC testing sample script loads the sample page shown below into the browser. If Chrome is running in headless mode, it is not visible on UI, but it usually opens like this in without headless mode. Since their is no desktop UI available on the native Linux OS server, headless mode can be used.
We use Selenium to test Ant Media Server streaming sample pages on a regular basis, as well as to run 24×7 WebRTC tests. Check out these sample scripts for sample pages and 24/7 tests.
You can also write your own scripts to do the webRTC testing with Selenium. Not only for WebRTC, you can do testing for other protocols as well, like RTMP, SRT, etc., using Python and its modules.
Conclusion
WebRTC testing using Selenium allows you to efficiently evaluate WebRTC streams, ensuring smooth performance and functionality. Furthermore, advanced options allow you to perform real-time load testing to simulate concurrent user interactions, evaluate system behavior under stress, and optimize stream quality for a better end-user experience.
If you have questions or need any support, contact us via a form, schedule a meeting to have a coffee and chat, or write directly to contact@antmedia.io so that we can democratize live streaming together.